Winform App On Mac Using Visual Studio
- Winform App On Mac Using Visual Studios
- Windows Form Application Visual Studio
- Winforms Visual Studio 2019
- Visual Studio Winforms Controls
- Visual Studio For Mac Winforms
- Winform App On Mac Using Visual Studio Windows 10
This article describes how to port your Windows Forms-based desktop app from .NET Framework to .NET Core 3.0 or later. The .NET Core 3.0 SDK includes support for Windows Forms applications. Windows Forms is still a Windows-only framework and only runs on Windows. This example uses the .NET Core SDK CLI to create and manage your project.
In this article, various names are used to identify types of files used for migration. When migrating your project, your files will be named differently, so mentally match them to the ones listed below:
File | Description |
---|---|
MyApps.sln | The name of the solution file. |
MyForms.csproj | The name of the .NET Framework Windows Forms project to port. |
MyFormsCore.csproj | The name of the new .NET Core project you create. |
MyAppCore.exe | The .NET Core Windows Forms app executable. |
Visual Studio for Mac.NET. Azure DevOps. Azure DevOps Server (TFS) 0. Visual Studio warnings for a Winform app if I close the app after starting to build the code again. Visual studio 2017 version 15.8 windows 10.0. Sylvia-List-ext reported Sep 19, 2018 at 12:02 PM. Jan 12, 2018 How about use NotifyIcon control in Winform application? Also please refer to the following documents: Doing a NotifyIcon program the right way. How to Show NotifyIcon in Windows Forms Application Using C#. Toast style popup for my application. Show Windows 10 toast from WinForm application. Send a local toast notification. Regards, Stanly.
Prerequisites
Visual Studio 2019 16.5 Preview 1 or later for any designer work you want to do. We recommend to update to the latest Preview version of Visual Studio.
Install the following Visual Studio workloads:
- .NET desktop development
- .NET Core cross-platform development
A working Windows Forms project in a solution that builds and runs without issue.
A project coded in C#.
Note
.NET Core 3.0 projects are only supported in Visual Studio 2019 or a later version. Starting with Visual Studio 2019 version 16.5 Preview 1, the .NET Core Windows Forms designer is also supported.
To enable the designer, go to Tools > Options > Environment > Preview Features and select the Use the preview Windows Forms designer for .NET Core apps option.
Consider
When porting a .NET Framework Windows Forms application, there are a few things you must consider.
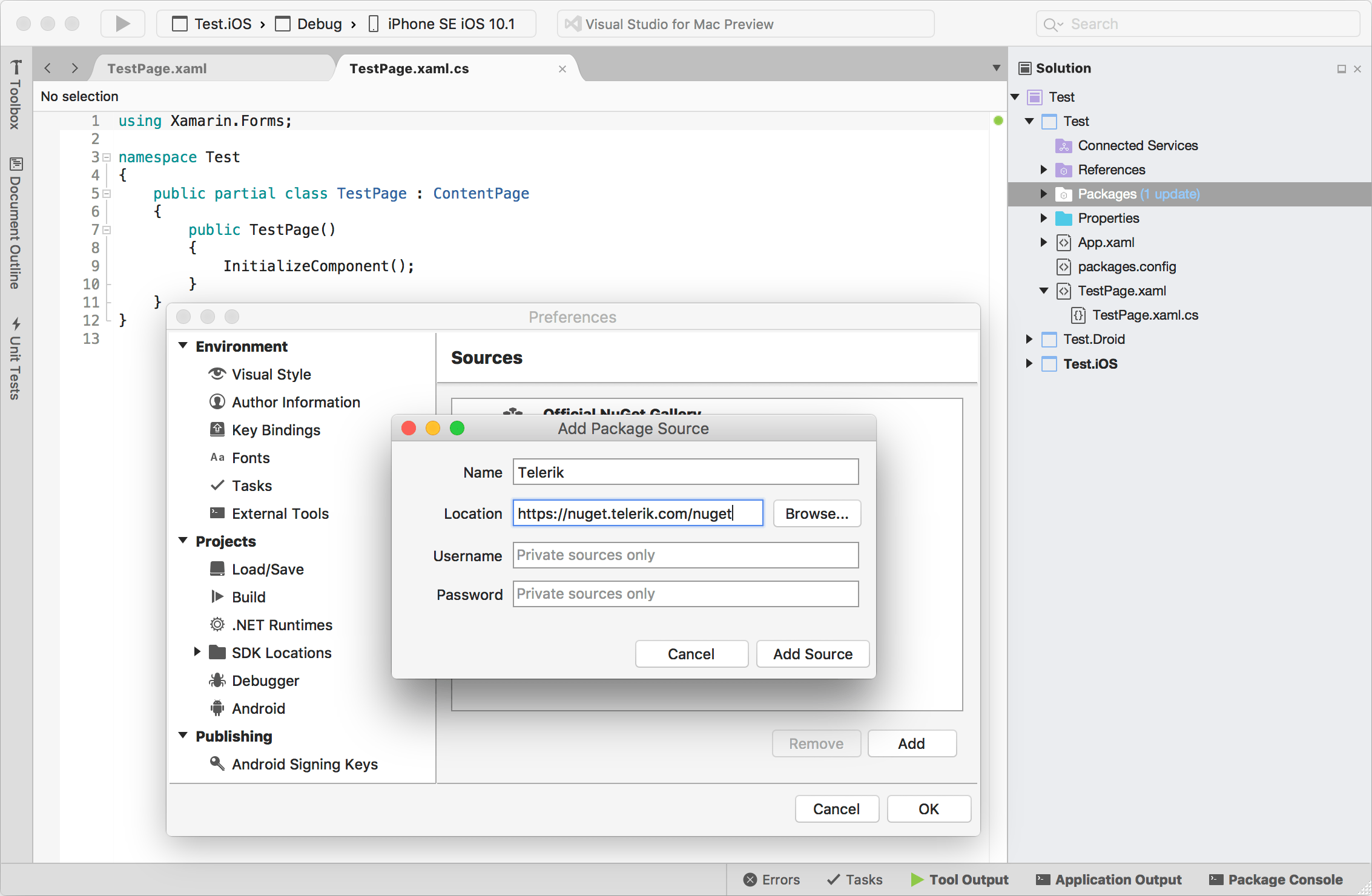
Check that your application is a good candidate for migration.
Use the .NET Portability Analyzer to determine if your project will migrate to .NET Core 3.0. If your project has issues with .NET Core 3.0, the analyzer helps you identify those problems.
You're using a different version of Windows Forms.
When .NET Core 3.0 Preview 1 was released, Windows Forms went open source on GitHub. The code for .NET Core Windows Forms is a fork of the .NET Framework Windows Forms codebase. It's possible some differences exist and your app won't port.
The Windows Compatibility Pack may help you migrate.
Some APIs that are available in .NET Framework aren't available in .NET Core 3.0. The Windows Compatibility Pack adds many of these APIs and may help your Windows Forms app become compatible with .NET Core.
Update the NuGet packages used by your project.
It's always a good practice to use the latest versions of NuGet packages before any migration. If your application is referencing any NuGet packages, update them to the latest version. Ensure your application builds successfully. After upgrading, if there are any package errors, downgrade the package to the latest version that doesn't break your code.
Create a new SDK project
The new .NET Core 3.0 project you create must be in a different directory from your .NET Framework project. If they're both in the same directory, you may run into conflicts with the files that are generated in the obj directory. In this example, we'll create a directory named MyFormsAppCore in the SolutionFolder directory:
Next, you need to create the MyFormsCore.csproj project in the MyFormsAppCore directory. You can create this file manually by using the text editor of choice. Paste in the following XML:
If you don't want to create the project file manually, you can use Visual Studio or the .NET Core SDK to generate the project. However, you must delete all other files generated by the project template except for the project file. To use the SDK, run the following command from the SolutionFolder directory:
After you create the MyFormsCore.csproj, your directory structure should look like the following:
Add the MyFormsCore.csproj project to MyApps.sln with either Visual Studio or the .NET Core CLI from the SolutionFolder directory:
Fix assembly info generation
Windows Forms projects that were created with .NET Framework include an AssemblyInfo.cs
file, which contains assembly attributes such as the version of the assembly to be generated. SDK-style projects automatically generate this information for you based on the SDK project file. Having both types of 'assembly info' creates a conflict. Resolve this problem by disabling automatic generation, which forces the project to use your existing AssemblyInfo.cs
file.
There are three settings to add to the main <PropertyGroup>
node.
GenerateAssemblyInfo
When you set this property tofalse
, it won't generate the assembly attributes. This avoids the conflict with the existingAssemblyInfo.cs
file from the .NET Framework project.AssemblyName
The value of this property is the output binary created when you compile. The name doesn't need an extension added to it. For example, usingMyCoreApp
producesMyCoreApp.exe
.RootNamespace
The default namespace used by your project. This should match the default namespace of the .NET Framework project.
Add these three elements to the <PropertyGroup>
node in the MyFormsCore.csproj
file:
Add source code
Right now, the MyFormsCore.csproj project doesn't compile any code. By default, .NET Core projects automatically include all source code in the current directory and any child directories. You must configure the project to include code from the .NET Framework project using a relative path. If your .NET Framework project used .resx files for icons and resources for your forms, you'll need to include those too.
Add the following <ItemGroup>
node to your project. Each statement includes a file glob pattern that includes child directories.
Alternatively, you can create a <Compile>
or <EmbeddedResource>
entry for each file in your .NET Framework project.
Add NuGet packages
Add each NuGet package referenced by the .NET Framework project to the .NET Core project.
Most likely your .NET Framework Windows Forms app has a packages.config file that contains a list of all of the NuGet packages that are referenced by your project. You can look at this list to determine which NuGet packages to add to the .NET Core project. For example, if the .NET Framework project referenced the MetroFramework
, MetroFramework.Design
, and MetroFramework.Fonts
NuGet packages, add each to the project with either Visual Studio or the .NET Core CLI from the SolutionFolder directory:
Winform App On Mac Using Visual Studios
The previous commands would add the following NuGet references to the MyFormsCore.csproj project:
Port control libraries
If you have a Windows Forms Controls library project to port, the directions are the same as porting a .NET Framework Windows Forms app project, except for a few settings. And instead of compiling to an executable, you compile to a library. The difference between the executable project and the library project, besides paths for the file globs that include your source code, is minimal.
Using the previous step's example, lets expand what projects and files we're working with.
File | Description |
---|---|
MyApps.sln | The name of the solution file. |
MyControls.csproj | The name of the .NET Framework Windows Forms Controls project to port. |
MyControlsCore.csproj | The name of the new .NET Core library project you create. |
MyCoreControls.dll | The .NET Core Windows Forms Controls library. |
Consider the differences between the MyControlsCore.csproj
project and the previously created MyFormsCore.csproj
project.
Here is an example of what the .NET Core Windows Forms Controls library project file would look like:
As you can see, the <OutputType>
node was removed, which defaults the compiler to produce a library instead of an executable. The <AssemblyName>
and <RootNamespace>
were changed. Specifically the <RootNamespace>
should match the namespace of the Windows Forms Controls library you are porting. And finally, the <Compile>
and <EmbeddedResource>
nodes were adjusted to point to the folder of the Windows Forms Controls library you are porting.
Next, in the main .NET Core MyFormsCore.csproj project, add a reference to the new .NET Core Windows Forms Control library. Add a reference with either Visual Studio or the .NET Core CLI from the SolutionFolder directory:
The previous command adds the following to the MyFormsCore.csproj project:
Compilation problems
If you have problems compiling your projects, you may be using some Windows-only APIs that are available in .NET Framework but not available in .NET Core. You can try adding the Windows Compatibility Pack NuGet package to your project. This package only runs on Windows and adds about 20,000 Windows APIs to .NET Core and .NET Standard projects.
The previous command adds the following to the MyFormsCore.csproj project:
Windows Forms Designer
As detailed in this article, Visual Studio 2019 only supports the Forms Designer in .NET Framework projects. By creating a side-by-side .NET Core project, you can test your project with .NET Core while you use the .NET Framework project to design forms. Your solution file includes both the .NET Framework and .NET Core projects. Add and design your forms and controls in the .NET Framework project, and based on the file glob patterns we added to the .NET Core projects, any new or changed files will automatically be included in the .NET Core projects.
Once Visual Studio 2019 supports the Windows Forms Designer, you can copy/paste the content of your .NET Core project file into the .NET Framework project file. Then delete the file glob patterns added with the <Source>
and <EmbeddedResource>
items. Fix the paths to any project reference used by your app. This effectively upgrades the .NET Framework project to a .NET Core project.
Next steps
- Learn about breaking changes from .NET Framework to .NET Core.
- Read more about the Windows Compatibility Pack.
- Watch a video on porting your .NET Framework Windows Forms project to .NET Core.
In this short introduction to the Visual Studio integrated development environment (IDE), you'll create a simple C# application that has a Windows-based user interface (UI).
Windows Form Application Visual Studio
If you haven't already installed Visual Studio, go to the Visual Studio downloads page to install it for free.
If you haven't already installed Visual Studio, go to the Visual Studio downloads page to install it for free.
Note
Some of the screenshots in this tutorial use the dark theme. If you aren't using the dark theme but would like to, see the Personalize the Visual Studio IDE and Editor page to learn how.
Create a project
First, you'll create a C# application project. The project type comes with all the template files you'll need, before you've even added anything.
Open Visual Studio 2017.
From the top menu bar, choose File > New > Project.
In the New Project dialog box in the left pane, expand Visual C#, and then choose Windows Desktop. In the middle pane, choose Windows Forms App (.NET Framework). Then name the file
HelloWorld
.If you don't see the Windows Forms App (.NET Framework) project template, cancel out of the New Project dialog box and from the top menu bar, choose Tools > Get Tools and Features. The Visual Studio Installer launches. Choose the .NET desktop development workload, then choose Modify.
Open Visual Studio 2019.
On the start window, choose Create a new project.
On the Create a new project window, choose the Windows Forms App (.NET Framework) template for C#.
(If you prefer, you can refine your search to quickly get to the template you want. For example, enter or type Windows Forms App in the search box. Next, choose C# from the Language list, and then choose Windows from the Platform list.)
Note
If you do not see the Windows Forms App (.NET Framework) template, you can install it from the Create a new project window. In the Not finding what you're looking for? message, choose the Install more tools and features link.
Next, in the Visual Studio Installer, choose the Choose the .NET desktop developmentCall center software for mac. workload.
After that, choose the Modify button in the Visual Studio Installer. You might be prompted to save your work; if so, do so. Next, choose Continue to install the workload. Then, return to step 2 in this 'Create a project' procedure.
In the Configure your new project window, type or enter HelloWorld in the Project name box. Then, choose Create.
Visual Studio opens your new project.
Create the application
After you select your C# project template and name your file, Visual Studio opens a form for you. A form is a Windows user interface. We'll create a 'Hello World' application by adding controls to the form, and then we'll run the app.
Add a button to the form
Choose Toolbox to open the Toolbox fly-out window.
(If you don't see the Toolbox fly-out option, you can open it from the menu bar. To do so, View > Toolbox. Or, press Ctrl+Alt+X.)
Choose the Pin icon to dock the Toolbox window.
Choose the Button control and then drag it onto the form.
In the Properties window, locate Text, change the name from Button1 to
Click this
, and then press Enter.(If you don't see the Properties window, you can open it from the menu bar. To do so, choose View > Properties Window. Or, press F4.)
In the Design section of the Properties window, change the name from Button1 to
btnClickThis
, and then press Enter.Note
If you've alphabetized the list in the Properties window, Button1 appears in the (DataBindings) section, instead.
Winforms Visual Studio 2019
Add a label to the form
Visual Studio Winforms Controls
Now that we've added a button control to create an action, let's add a label control to send text to.
Select the Label control from the Toolbox window, and then drag it onto the form and drop it beneath the Click this button.
In either the Design section or the (DataBindings) section of the Properties window, change the name of Label1 to
lblHelloWorld
, and then press Enter.
Visual Studio For Mac Winforms
Add code to the form
In the Form1.cs [Design] window, double-click the Click this button to open the Form1.cs window.
(Alternatively, you can expand Form1.cs in Solution Explorer, and then choose Form1.)
In the Form1.cs window, after the private void line, type or enter
lblHelloWorld.Text = 'Hello World!';
as shown in the following screenshot:
Winform App On Mac Using Visual Studio Windows 10
Run the application
Choose the Start button to run the application.
Several things will happen. In the Visual Studio IDE, the Diagnostics Tools window will open, and an Output window will open, too. But outside of the IDE, a Form1 dialog box appears. It will include your Click this button and text that says Label1.
Choose the Click this button in the Form1 dialog box. Notice that the Label1 text changes to Hello World!.
Close the Form1 dialog box to stop running the app.
Next steps
To learn more, continue with the following tutorial: